ThreadLock Demystified: A Deep Dive into Thread Synchronization
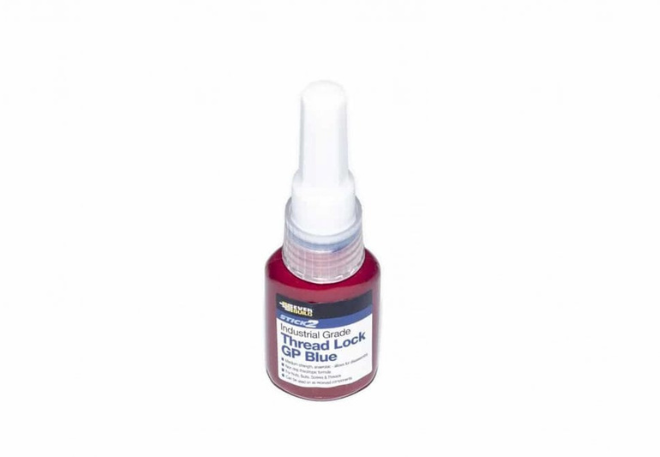
Multithreading is a powerful tool in the hands of developers. It allows for the concurrent execution of tasks, providing the potential to maximize CPU usage and optimize application responsiveness. However, with this power comes the inherent challenge of concurrency issues, such as race conditions. This is where thread synchronization tools like ThreadLock come into play. Let’s demystify the often perplexing world of ThreadLock and explore its critical role in achieving seamless thread synchronization.
Understanding Threads and the Need for Synchronization
Before diving deep into ThreadLock, it’s essential to understand why we need it in the first place.
Threads: In the realm of computer programming, a thread is the smallest sequence of programmed instructions that can be managed independently by an OS scheduler. Essentially, threads allow parts of an application to run concurrently.
Concurrency Issues: When two or more threads access shared resources concurrently, they can produce unpredictable results. This problem, known as a race condition, occurs when the behavior of an application depends on the relative timing of events.
To prevent race conditions and ensure that only one thread can access a shared resource at a time, we need synchronization mechanisms. Enter the world of locks.
ThreadLock: What is it?
A ThreadLock, often just called a “lock”, is a synchronization primitive. At its core, it acts like a gatekeeper. When a thread wishes to access a protected section of code, it must first acquire the lock. If another thread already holds the lock, the requesting thread must wait. Once the lock is released, it can be acquired by another thread.
The primary purpose of a ThreadLock is to ensure that only one thread can execute a specific section of the code at any given time.
How does ThreadLock Work?
Acquisition and Release: The core functions associated with a lock are the acquisition and release operations. When a thread attempts to acquire a lock that’s already held, it will block (or wait) until the lock is released. Post release, the waiting thread can then acquire it.
Reentrancy: Some locks are ‘reentrant’, meaning that if a thread holds a lock, it can acquire it again without blocking. This feature prevents a thread from deadlocking with itself. Not all locks are reentrant, but those that are can be especially useful in certain situations.
Common Pitfalls and Best Practices
While ThreadLock offers a straightforward approach to thread synchronization, it’s not without its challenges:
Deadlocks: This is when two or more threads are unable to proceed with their tasks because they are each waiting for the other to release locks. For instance, if Thread A holds Lock 1 and waits for Lock 2, while Thread B holds Lock 2 and waits for Lock 1, a deadlock occurs. Both threads are stuck indefinitely.
Starvation: This is a situation where a thread is perpetually denied access to a lock because other threads are always ahead of it in the queue. Over time, this can seriously degrade performance.
Lock contention: When several threads frequently compete for a lock, it can lead to performance bottlenecks.
To avoid these issues, keep the following best practices in mind:
- Minimize Lock Duration: Hold locks only as long as necessary. The shorter the duration, the less likely you’ll run into problems like contention.
- Avoid Nested Locks: If you must acquire multiple locks, always acquire them in a consistent order.
- Use Timed Locks: Where possible, use locks that can time out, which can prevent indefinite deadlocks.
- Consider Higher-level Synchronization: Sometimes, other synchronization mechanisms, like semaphores or conditions, might be more appropriate.
Final Thoughts
ThreadLock is a foundational tool in the arsenal of a multithreaded application developer. It provides a straightforward way to protect shared resources from race conditions. By understanding its workings and adhering to best practices, developers can harness the power of multithreading while minimizing potential pitfalls.
Remember, like all tools, ThreadLock is just a means to an end. Always consider the specific needs of your application and whether other synchronization mechanisms might be more appropriate. With a clear understanding and a judicious approach, seamless thread synchronization is within reach.